ASP.NET Core 2.0 초기 매개 변 수 를 가 진 미들웨어 문제 및 해결 방법
어떻게 ASP.NET Core 2.0 에서 미들웨어 에 초기 인 자 를 전달 합 니까?
답안
빈 항목 에서 중간 에 필요 한 인 자 를 저장 하기 위해 POCO(Plain Old CLR Object)를 만 듭 니 다.
public class GreetingOptions
{
public string GreetAt { get; set; }
public string GreetTo { get; set; }
}
미들웨어 추가:
public class GreetingMiddleware
{
private readonly RequestDelegate _next;
private readonly GreetingOptions _options;
public GreetingMiddleware(RequestDelegate next, GreetingOptions options)
{
_next = next;
_options = options;
}
public async Task Invoke(HttpContext context)
{
var message = $"Good {_options.GreetAt} {_options.GreetTo}";
await context.Response.WriteAsync(message);
}
}
답 1:실례 유형미들웨어 를 설정 하기 위해 확장 방법 을 추가 합 니 다:
public static IApplicationBuilder UseGreetingMiddleware(this IApplicationBuilder app, GreetingOptions options)
{
return app.UseMiddleware<GreetingMiddleware>(options);
}
중간 부품 사용 하기:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseGreetingMiddleware(new GreetingOptions {
GreetAt = "Morning",
GreetTo = "Tahir"
});
}
정 답 2:함수 유형미들웨어 를 설정 하기 위해 확장 방법 을 추가 합 니 다:
public static IApplicationBuilder UseGreetingMiddlewareAction(this IApplicationBuilder app, Action<GreetingOptions> optionsAction)
{
var options = new GreetingOptions();
optionsAction(options);
return app.UseMiddleware<GreetingMiddleware>(options);
}
중간 부품 사용 하기:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseGreetingMiddlewareAction(options =>
{
options.GreetAt = "Morning";
options.GreetTo = "Tahir";
});
}
상술 한 두 가지 방법 은 결과 가 일치한다.실행,이 때 페이지 표시:
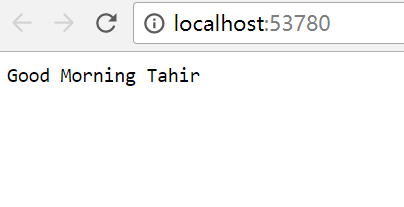
토론 하 다.
이전에 우 리 는 단독 클래스 에서 중간 부품 을 정의 하고 확장 방법 을 사용 하여 요청 파이프 에 추가 하 는 것 이 가장 좋 은 실천 이 라 고 토론 한 적 이 있다.우 리 는 중간물 에 인 자 를 전달 해 야 할 수도 있 습 니 다.ASP.NET 코어 소스 코드 와 다른 온라인 예제 에 대한 학습 을 통 해 저 는 상기 두 가지 모델 을 정리 해 냈 습 니 다.
상술 한 두 가지 해결 방법 은 모두 매우 직관 적 이다.우 리 는 파 라 메 터 를 하나의 POCO 클래스 에 밀봉 한 다음 확장 방법 을 만들어 다음 파 라 메 터 를 받 아들 입 니 다.
1.POCO 실례
2.호출 할 함수(함수 에 POCO 설정)
주:POCO 인 스 턴 스 는 구조 함 수 를 통 해 미들웨어 로 전 달 됩 니 다.UseMiddleware()방법 은 가 변 매개 변수 params object[]를 수신 하고 이 매개 변 수 를 미들웨어 구조 함수 에 전달 합 니 다.
서비스 설정
이 모드 들 은 서비스 용기 에 서비스 인 스 턴 스 를 추가 하 는 데 도 사용 할 수 있다.설명 하기 편리 하도록 우 리 는 먼저 서 비 스 를 추가 합 니 다.
public interface IMessageService
{ string FormatMessage(string message);
}
public class MessageService : IMessageService
{
private readonly GreetingOptions _options;
public MessageService(GreetingOptions options)
{
_options = options;
}
public string FormatMessage(string message)
{
return $"Good {_options.GreetAt} {_options.GreetTo} - {message}";
}
}
서 비 스 를 설정 하기 위해 다음 확장 방법 을 추가 합 니 다:
public static IServiceCollection AddMessageService(this IServiceCollection services, GreetingOptions options)
{ return services.AddScoped<IMessageService>(factory => new MessageService(options));
}
public static IServiceCollection AddMessageServiceAction(this IServiceCollection services, Action<GreetingOptions> optionsAction)
{
var options = new GreetingOptions();
optionsAction(options);
return services.AddScoped<IMessageService>(factory => new MessageService(options));
}
Configure()에서 이 서 비 스 를 사용 합 니 다:
public void ConfigureServices(IServiceCollection services)
{
services.AddMessageService(new GreetingOptions
{
GreetAt = "Morning",
GreetTo = "Tahir"
});
services.AddMessageServiceAction(options =>
{
options.GreetAt = "Morning";
options.GreetTo = "Tahir";
});
}
Configure Services()가 Configure()보다 먼저 실행 되 기 때문에 Configure()에 이 서 비 스 를 직접 주입 할 수 있 습 니 다.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, IMessageService msg)
{
app.Run(async (context) =>
{
await context.Response.WriteAsync(msg.FormatMessage("by sanshi"));
});
}
실행,이 때 페이지 표시: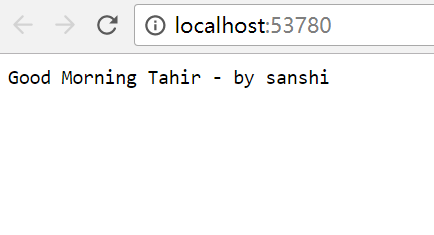
클릭 하여 원본 다운로드:http://xiazai.jb51.net/201710/yuanma/MiddlewareWithParameters.rar
총결산
위 에서 말 한 것 은 소 편 이 여러분 에 게 소개 한 ASP.NET Core 2.0 의 초기 매개 변 수 를 가 진 미들웨어 문제 와 해결 방법 입 니 다.여러분 에 게 도움 이 되 기 를 바 랍 니 다.궁금 한 점 이 있 으 시 면 메 시 지 를 남 겨 주세요.소 편 은 신속하게 답 해 드 리 겠 습 니 다.여기 서도 저희 사이트 에 대한 여러분 의 지지 에 감 사 드 립 니 다!
이 내용에 흥미가 있습니까?
현재 기사가 여러분의 문제를 해결하지 못하는 경우 AI 엔진은 머신러닝 분석(스마트 모델이 방금 만들어져 부정확한 경우가 있을 수 있음)을 통해 가장 유사한 기사를 추천합니다:
AS를 통한 Module 개발1. ModuleLoader 사용 2. IModuleInfo 사용 ASModuleOne 모듈...
텍스트를 자유롭게 공유하거나 복사할 수 있습니다.하지만 이 문서의 URL은 참조 URL로 남겨 두십시오.
CC BY-SA 2.5, CC BY-SA 3.0 및 CC BY-SA 4.0에 따라 라이센스가 부여됩니다.