jQuery를 사용하여 업로드하기 전에 이미지 미리보기
3338 단어 imageuploadhtmljquerywebdev
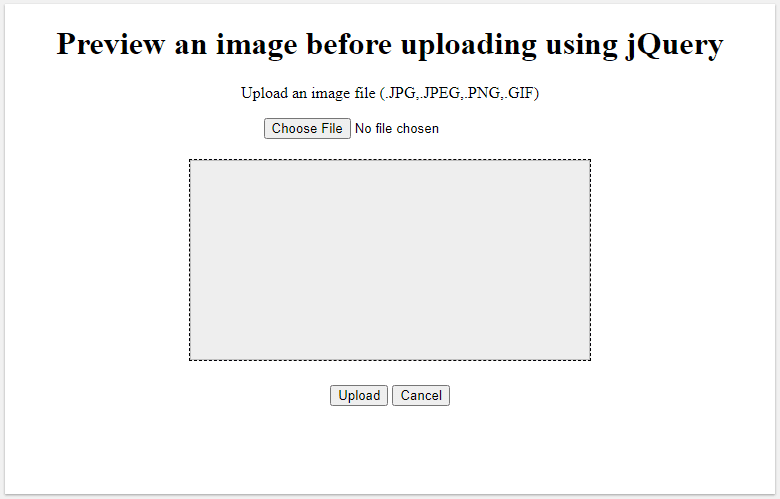
이미지를 서버에 업로드하기 전에 미리 보거나 표시하는 것은 최상의 사용자 경험을 제공하는 것입니다.
여기서는 HTML, CSS 및 jQuery를 사용하여 개발할 것입니다.
아래의 간단한 단계를 따르기만 하면 됩니다.
HTML
<head>
<!-- jQuery library script import -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<h1>Preview an image before uploading using jQuery</h1>
<p>Upload an image file (.JPG,.JPEG,.PNG,.GIF) </p>
<!-- input element to choose a file for uploading -->
<input type="file" accept="image/*" id="image-upload" />
<br>
<!-- img element to preview or display the uploading image -->
<img id="image-container" />
<br>
<button id="upload-btn" >Upload</button>
<button id="cancel-btn" >Cancel</button>
</body>
CSS
/* to make everything center aligned */
body{
text-align:center;
}
/* to set specific height and width for image preview */
#image-container{
margin : 20px;
height: 200px;
width: 400px;
background-color:#eee;
border: 1px dashed #000;
}
jQuery 코드
/* This function will call when page loaded successfully */
$(document).ready(function(){
/* This function will call when onchange event fired */
$("#image-upload").on("change",function(){
/* Current this object refer to input element */
var $input = $(this);
var reader = new FileReader();
reader.onload = function(){
$("#image-container").attr("src", reader.result);
}
reader.readAsDataURL($input[0].files[0]);
});
/* This function will call when upload button clicked */
$("#upload-btn").on("click",function(){
/* file validation logic goes here if required */
/* image uploading logic goes here */
alert("Upload logic need to be write here...");
});
/* This function will call when cancel button clicked */
$("#cancel-btn").on("click",function(){
/* Reset input element */
$('#image-upload').val("");
/* Clear image preview container */
$('#image-container').attr("src","");
});
});
여기 기사에서 자세한 설명을 알아보세요: Preview an image before uploading using jQuery
Reference
이 문제에 관하여(jQuery를 사용하여 업로드하기 전에 이미지 미리보기), 우리는 이곳에서 더 많은 자료를 발견하고 링크를 클릭하여 보았다 https://dev.to/javacodepoint/preview-an-image-before-uploading-using-jquery-4emc텍스트를 자유롭게 공유하거나 복사할 수 있습니다.하지만 이 문서의 URL은 참조 URL로 남겨 두십시오.
우수한 개발자 콘텐츠 발견에 전념
(Collection and Share based on the CC Protocol.)