Springboot+Netty+Websocket 메시지 전송 인 스 턴 스 구현
14010 단어 SpringbootWebsocket메시지 푸 시
WebSocket 은 클 라 이언 트 와 서버 간 의 데이터 교환 을 더욱 간단하게 하고 서버 가 자발적으로 클 라 이언 트 에 데 이 터 를 전송 할 수 있 도록 합 니 다.웹 소켓 API 에 서 는 브 라 우 저 와 서버 가 악 수 를 한 번 만 하면 영구적 인 연결 을 만 들 고 양 방향 데이터 전송 을 할 수 있다.
Netty 프레임 워 크 의 장점
1.API 사용 이 간단 하고 개발 문턱 이 낮다.
2.기능 이 강하 고 여러 가지 디 코딩 기능 을 미리 설정 하여 다양한 주류 협 의 를 지원 합 니 다.
3.맞 춤 형 능력 이 강하 고 Channel Handler 를 통 해 통신 구 조 를 유연 하 게 확장 할 수 있다.
4.성능 이 높 고 다른 업계 주류 의 NIO 프레임 워 크 와 비 교 를 통 해 Netty 의 종합 성능 이 가장 좋다.
5.성숙 하고 안정 적 인 Netty 는 이미 발 견 된 모든 JDK NIO BUG 를 복 구 했 습 니 다.업무 개발 자 들 은 NIO BUG 에 대해 더 이상 고민 할 필요 가 없습니다.
제시:다음은 본 글 의 본문 내용 이 고 다음 사례 는 참고 할 수 있 습 니 다.
넷 티 의존 도입
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.48.Final</version>
</dependency>
2.사용 절차1.기본 설정 클래스 도입
package com.test.netty;
public enum Cmd {
START("000", " "),
WMESSAGE("001", " "),
;
private String cmd;
private String desc;
Cmd(String cmd, String desc) {
this.cmd = cmd;
this.desc = desc;
}
public String getCmd() {
return cmd;
}
public String getDesc() {
return desc;
}
}
2.netty 서비스 시작 모니터
package com.test.netty;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.ApplicationRunner;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
/**
* @author test
* <p>
*
**/
@Slf4j
@Component
public class NettyServer {
@Value("${server.netty.port}")
private int port;
@Autowired
private ServerChannelInitializer serverChannelInitializer;
@Bean
ApplicationRunner nettyRunner() {
return args -> {
//new
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
//new
EventLoopGroup workGroup = new NioEventLoopGroup();
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workGroup)
.channel(NioServerSocketChannel.class)
.childHandler(serverChannelInitializer)
//
.option(ChannelOption.SO_BACKLOG, 1024)
// ,TCP
.childOption(ChannelOption.SO_KEEPALIVE, true);
// ,
try {
ChannelFuture future = bootstrap.bind(port).sync();
log.info(" : {}", port);
future.channel().closeFuture().sync();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
//
bossGroup.shutdownGracefully();
//
workGroup.shutdownGracefully();
}
};
}
}
3.netty 서버 프로세서
package com.test.netty;
import com.test.common.util.JsonUtil;
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
import lombok.Data;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.net.URLDecoder;
import java.util.*;
/**
* @author test
* <p>
* netty
**/
@Slf4j
@Component
@ChannelHandler.Sharable
public class NettyServerHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
@Autowired
private ServerChannelCache cache;
private static final String dataKey = "test=";
@Data
public static class ChannelCache {
}
/**
*
*/
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
Channel channel = ctx.channel();
log.info(" ,ID->{}......", channel.id().asLongText());
}
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if (evt instanceof WebSocketServerProtocolHandler.HandshakeComplete) {
Channel channel = ctx.channel();
WebSocketServerProtocolHandler.HandshakeComplete handshakeComplete = (WebSocketServerProtocolHandler.HandshakeComplete) evt;
String requestUri = handshakeComplete.requestUri();
requestUri = URLDecoder.decode(requestUri, "UTF-8");
log.info("HANDSHAKE_COMPLETE,ID->{},URI->{}", channel.id().asLongText(), requestUri);
String socketKey = requestUri.substring(requestUri.lastIndexOf(dataKey) + dataKey.length());
if (socketKey.length() > 0) {
cache.add(socketKey, channel);
this.send(channel, Cmd.DOWN_START, null);
} else {
channel.disconnect();
ctx.close();
}
}
super.userEventTriggered(ctx, evt);
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
Channel channel = ctx.channel();
log.info(" ,ID->{}, ID->{}......", channel.id().asLongText(), cache.getCacheId(channel));
cache.remove(channel);
}
/**
*
*/
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
Channel channel = ctx.channel();
log.error(" ,ID->{}, ID->{}, ->{}......", channel.id().asLongText(), cache.getCacheId(channel), cause.getMessage(), cause);
cache.remove(channel);
ctx.close();
}
/**
*
*/
@Override
protected void channelRead0(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
try {
// log.info(" :{}", msg.text());
ctx.channel().writeAndFlush(new TextWebSocketFrame(JsonUtil.toString(Collections.singletonMap("cmd", "100"))));
} catch (Exception e) {
log.error(" :{}", e.getMessage(), e);
}
}
public void send(Cmd cmd, String id, Object obj) {
HashMap<String, Channel> channels = cache.get(id);
if (channels == null) {
return;
}
Map<String, Object> data = new LinkedHashMap<>();
data.put("cmd", cmd.getCmd());
data.put("data", obj);
String msg = JsonUtil.toString(data);
log.info(" : {}", msg);
channels.values().forEach(channel -> {
channel.writeAndFlush(new TextWebSocketFrame(msg));
});
}
public void send(Channel channel, Cmd cmd, Object obj) {
Map<String, Object> data = new LinkedHashMap<>();
data.put("cmd", cmd.getCmd());
data.put("data", obj);
String msg = JsonUtil.toString(data);
log.info(" : {}", msg);
channel.writeAndFlush(new TextWebSocketFrame(msg));
}
}
4.netty 서버 캐 시 클래스
package com.test.netty;
import io.netty.channel.Channel;
import io.netty.util.AttributeKey;
import org.springframework.stereotype.Component;
import java.util.HashMap;
import java.util.concurrent.ConcurrentHashMap;
@Component
public class ServerChannelCache {
private static final ConcurrentHashMap<String, HashMap<String, Channel>> CACHE_MAP = new ConcurrentHashMap<>();
private static final AttributeKey<String> CHANNEL_ATTR_KEY = AttributeKey.valueOf("test");
public String getCacheId(Channel channel) {
return channel.attr(CHANNEL_ATTR_KEY).get();
}
public void add(String cacheId, Channel channel) {
channel.attr(CHANNEL_ATTR_KEY).set(cacheId);
HashMap<String, Channel> hashMap = CACHE_MAP.get(cacheId);
if (hashMap == null) {
hashMap = new HashMap<>();
}
hashMap.put(channel.id().asShortText(), channel);
CACHE_MAP.put(cacheId, hashMap);
}
public HashMap<String, Channel> get(String cacheId) {
if (cacheId == null) {
return null;
}
return CACHE_MAP.get(cacheId);
}
public void remove(Channel channel) {
String cacheId = getCacheId(channel);
if (cacheId == null) {
return;
}
HashMap<String, Channel> hashMap = CACHE_MAP.get(cacheId);
if (hashMap == null) {
hashMap = new HashMap<>();
}
hashMap.remove(channel.id().asShortText());
CACHE_MAP.put(cacheId, hashMap);
}
}
5.netty 서비스 초기 화
package com.test.netty;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
import io.netty.handler.stream.ChunkedWriteHandler;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
/**
* @author test
* <p>
* netty
**/
@Component
public class ServerChannelInitializer extends ChannelInitializer<SocketChannel> {
@Autowired
private NettyServerHandler nettyServerHandler;
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception {
ChannelPipeline pipeline = socketChannel.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new HttpObjectAggregator(8192));
pipeline.addLast(new WebSocketServerProtocolHandler("/test.io", true, 5000));
pipeline.addLast(nettyServerHandler);
}
}
6.html 테스트
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>test</title>
<script type="text/javascript">
function WebSocketTest()
{
if ("WebSocket" in window)
{
alert(" WebSocket!");
// web socket
var ws = new WebSocket("ws://localhost:port/test.io");
ws.onopen = function()
{
// Web Socket , send()
ws.send(" ");
alert(" ...");
};
ws.onmessage = function (evt)
{
var received_msg = evt.data;
alert(" ...");
};
ws.onclose = function()
{
// websocket
alert(" ...");
};
}
else
{
// WebSocket
alert(" WebSocket!");
}
}
</script>
</head>
<body>
<div id="sse">
<a href="javascript:WebSocketTest()" rel="external nofollow" > WebSocket</a>
</div>
</body>
</html>
7.vue 테스트
mounted() {
this.initWebsocket();
},
methods: {
initWebsocket() {
let websocket = new WebSocket('ws://localhost:port/test.io?test=123456');
websocket.onmessage = (event) => {
let msg = JSON.parse(event.data);
switch (msg.cmd) {
case "000":
this.$message({
type: 'success',
message: " !",
duration: 1000
})
setInterval(()=>{websocket.send("heartbeat")},60*1000);
break;
case "001":
this.$message.warning(" , !")
break;
}
}
websocket.onclose = () => {
setTimeout(()=>{
this.initWebsocket();
},30*1000);
}
websocket.onerror = () => {
setTimeout(()=>{
this.initWebsocket();
},30*1000);
}
},
},
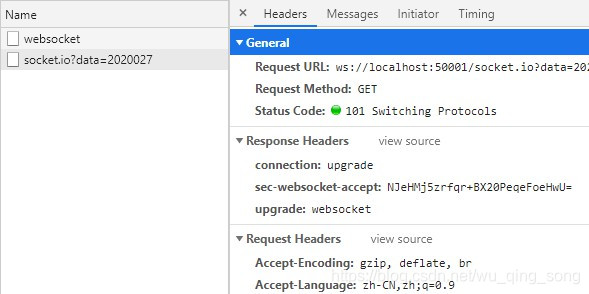
8.서버 에서 메시지 보 내기
@Autowired
private NettyServerHandler nettyServerHandler;
nettyServerHandler.send(CmdWeb.WMESSAGE, id, message);
Springboot+Netty+Websocket 구현 메시지 푸 시 인 스 턴 스 에 관 한 이 글 은 여기까지 소개 되 었 습 니 다.Springboot Websocket 메시지 푸 시 내용 은 이전 글 을 검색 하거나 아래 의 관련 글 을 계속 찾 아 보 세 요.앞으로 많은 응원 부탁드립니다!
이 내용에 흥미가 있습니까?
현재 기사가 여러분의 문제를 해결하지 못하는 경우 AI 엔진은 머신러닝 분석(스마트 모델이 방금 만들어져 부정확한 경우가 있을 수 있음)을 통해 가장 유사한 기사를 추천합니다:
[MeU] Hashtag 기능 개발➡️ 기존 Tag 테이블에 존재하지 않는 해시태그라면 Tag , tagPostMapping 테이블에 모두 추가 ➡️ 기존에 존재하는 해시태그라면, tagPostMapping 테이블에만 추가 이후에 개발할 태그 기반 ...
텍스트를 자유롭게 공유하거나 복사할 수 있습니다.하지만 이 문서의 URL은 참조 URL로 남겨 두십시오.
CC BY-SA 2.5, CC BY-SA 3.0 및 CC BY-SA 4.0에 따라 라이센스가 부여됩니다.